HTTP Status Code 496 – SSL Certificate Required
The HTTP status code 496 SSL Certificate Required is a non-standard status code, used by some web servers to indicate that a client must provide a valid SSL certificate for completing the request. This code is typically used in scenarios where secure communication is mandatory, and the server needs to authenticate the client’s identity using SSL/TLS protocols.
Examples
Example 1: Nginx Configuration
In Nginx, you can configure your server to require SSL client certificates by using the ssl_verify_client
directive. If a client attempts to connect without providing a certificate, Nginx can return a 496 status code.
server {
listen 443 ssl;
server_name example.com;
ssl_certificate /path/to/server.crt;
ssl_certificate_key /path/to/server.key;
ssl_client_certificate /path/to/ca.crt;
ssl_verify_client on;
# Return 496 when client certificate is missing
error_page 496 = /496.html;
location / {
try_files $uri $uri/ =404;
}
location = /496.html {
internal;
return 496 "SSL Certificate Required";
}
}
In this configuration, Nginx listens on port 443 for HTTPS connections. It requires a client certificate by setting ssl_verify_client on;
. If a client does not provide a valid certificate, the request is redirected to a custom error page that returns a 496 status code.
Example 2: Handling 496 Status Code in Code
If you’re developing an application that interacts with an API requiring client certificates, you must handle the 496 status code appropriately. Here’s an example using Python’s requests
library:
import requests
url = 'https://api.example.com/data'
# Path to client certificate and key
client_cert = ('/path/to/client.crt', '/path/to/client.key')
try:
response = requests.get(url, cert=client_cert)
response.raise_for_status()
# Process the response if the certificate is valid
print(response.json())
except requests.exceptions.SSLError as e:
# Handle the SSL error (could be a 496 error)
print("SSL Certificate Required or invalid certificate:", e)
except requests.exceptions.HTTPError as e:
if e.response.status_code == 496:
print("SSL Certificate Required:", e.response.text)
else:
print("HTTP error occurred:", e)
In this Python example, the requests
library is used to make an HTTPS request to an API that requires a client certificate. The cert
parameter is used to specify the path to the client certificate and key. If the server returns a 496 status code, it indicates that the request is missing a valid client certificate.
Example 3 Scenario
# Client sends a request example. GET /example HTTP/1.1 Host: www.example.com # Server Response HTTP/1.1 496 496 SSL Certificate Required Date: Wed, 09 Oct 2024 23:09:19 GMT Server: ExampleServer/1.0 Content-Type: application/json { "error": "Description of the error for 496" }
Example 4 Scenario
# Client sends another example request. POST /another-example HTTP/1.1 Host: www.example.com # Server Response HTTP/1.1 496 496 SSL Certificate Required Date: Wed, 09 Oct 2024 23:09:19 GMT Server: ExampleServer/1.0 Content-Type: application/json { "error": "Detailed message for 496" }
Summary
The 496 SSL Certificate Required status code is used to enforce SSL client authentication. It ensures that clients provide a valid certificate for secure communication and authentication. This status code is non-standard and primarily used in specific server configurations like Nginx. When implementing client-server communication requiring SSL certificates, it’s essential to handle this status code to ensure secure interactions.
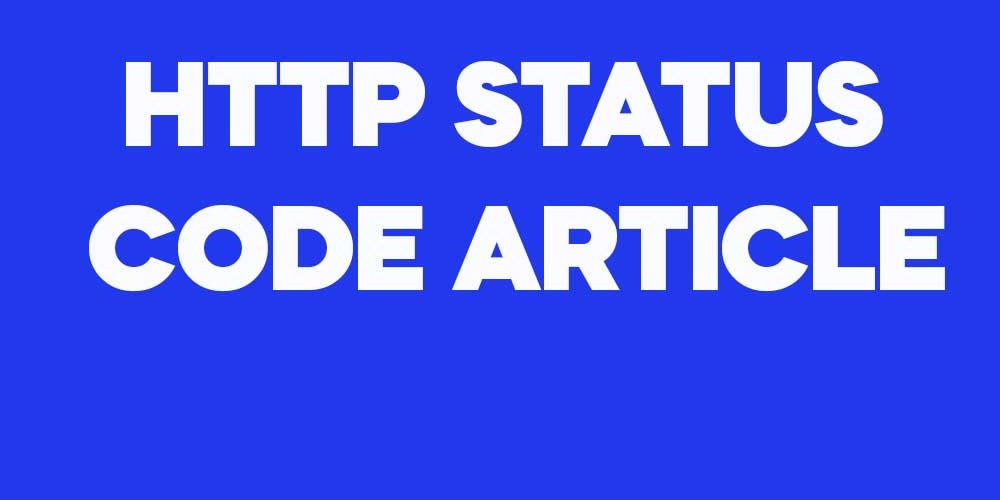